Backend Development
Master Java Backend Development with Industry Experts
Looking to build a career as a Java Backend Developer? Our Java Backend Certification from Techtroma is designed to equip you with the essential skills required to develop scalable, high-performance backend systems. Learn from industry experts and get hands-on experience with Spring Boot, REST APIs, Microservices, Database Management, and Cloud Deployment.
Why Choose Our Java Backend Certification?
- Industry-Aligned Curriculum – Learn core backend development concepts with real-world applications.
- Hands-on Projects – Work on live projects to gain practical exposure.
- Expert-Led Training – Learn from experienced Java professionals.
- Certification from Techtroma – A recognized certification to boost your career prospects.
- Placement Assistance – Get guidance on resume building, interview preparation, and job referrals.
What You Will Learn?
- Core Java and Object-Oriented Programming (OOPs)
- Advanced Java Concepts (Collections, Multithreading, Exception Handling)
- Spring Framework & Spring Boot
- Building RESTful APIs and Microservices
- Database Integration (MySQL, PostgreSQL)
- Deployment on AWS and DevOps Basics
- Secure Coding Practices and Performance Optimization
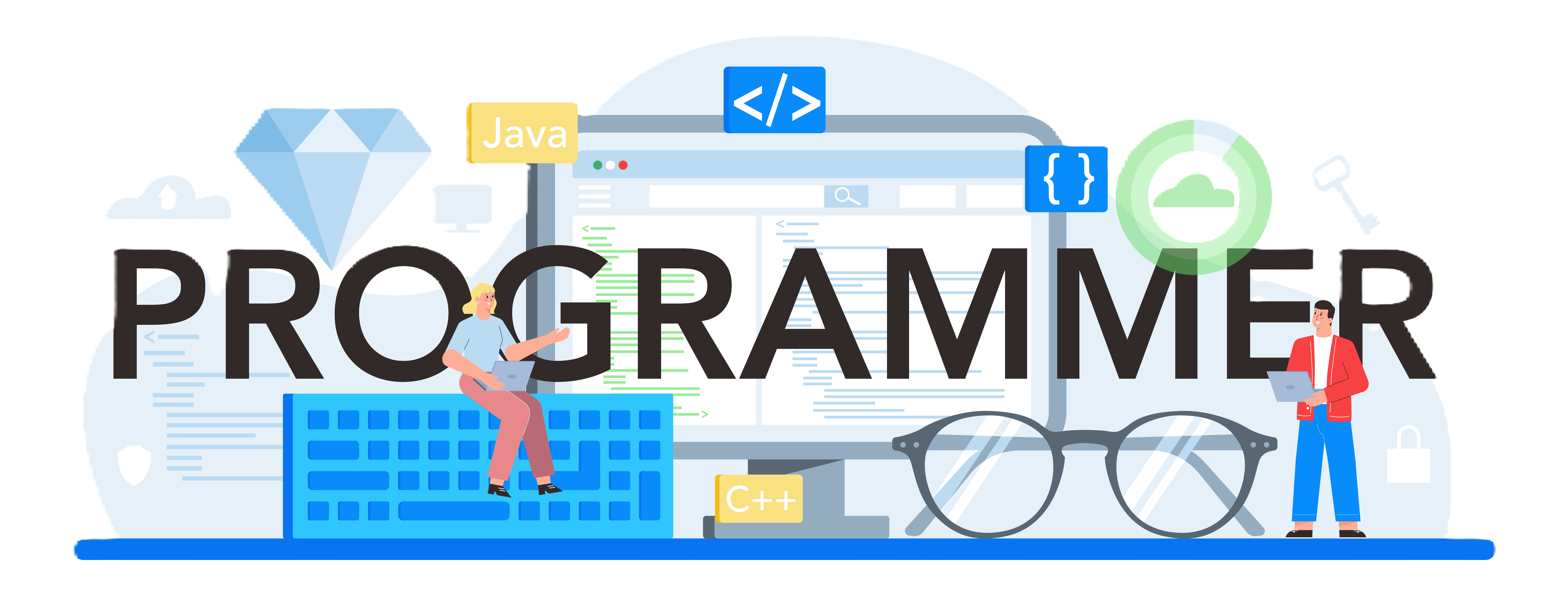
Who Can Enroll?
Beginners & Freshers – Want to start a career in backend development? This course is for you!
Software Developers – Looking to upskill in Java backend development?
IT Professionals – Want to switch to a backend development role?
Certification & Career Opportunities
Upon successful completion, you will receive a Java Backend Development Certification from Techtroma, opening doors to exciting roles such as:
- Java Backend Developer
- Software Engineer
- API Developer
- Cloud & DevOps Engineer
Course Curriculum
1.Introduction to Java
1.1 Introduction to Java
1.2 What is Java?
1.3 Setting up JDK.
1.4 Installing Java In Visual Studio Code.
1.5 Writing the first Java Program
2.Setting up JDK
2.1 How to setup JDK in visual studio code
2.2 Understanding the structure of a Java Project.
2.3 Building a web application from scratch.
3.Java Programming - Syntax
3.1 Introduction
3.2 Variables- int, Number, Boolean
3.3 String, List and set
3.4 String Operation and Functions.
3.5 Map operation and Functions.
3.6 List operation and Functions
3.7 Set operation and Functions.
3.8 Hash operation and Functions.
3.9 If-else, elif ,Statement
3.10 Loops & Break and Continue Statement..
4.Functions
4.1 Function Structure
4.2 Creating a Function
4.3 Function Return Data Types
4.4 Lambda Function
5.Annotations
5.1 Annotations basics
5.2 Declaring an annotation type
5.3 Predefined annotation types
5.4 Repeating annotations
5.5 Type annotations and pluggable type system
6.Modules
6.1 Introduction to Modules.
6.2 Importing Modules.
6.3 Syntax for importing Modules and Functionality.
6.4 Learning Goals.
6.5 Objectives.
7.Conditional Control Statement
7.1 If Statement.
7.2 If-else Statement.
7.3 If-else-if statement.
7.4 Nested if Statement.
7.5 Switch statement.
8.Looping Statement.
8.1 For loop.
8.2 While-loop.
8.3 Pass Statement.
8.4 Break Statement.
8.5 Continue Statement.
9.Type Conversion.
9.1 Introduction .
9.2 Overview.
9.3 Types of Type Conversion.
9.4 Implicit 9.5 Explicit
10.Java Operators.
10.1 Arithmetic Operator.
10.2 Comparison operator.
10.3 Logical operator. 10.4 Shift operator.
10.4 Bitwise operator.
10.5 Ternary operator.
10.6 Unary operators.
10.7 Examples.
11.Operator overloading.
11.1 Introduction.
11.2 Method Overloading.
11.3 Method Overriding.
12.Access Specifier.
12.1 Private Class access Specifier.
12.2 Public Class access Specifier.
12.3 Protected Class access Specifier
12.4 Default Class access Specifier.
13.Exception Handling
13.1 What is an Exception.
13.2 Exception Handling flow-of-control.
13.3 When to use try/except.
13.4 Raising and catching errors.
14.Spring Framework
Using Spring Frame work we can built web application.
15.Object-Oriented Concepts.
15.1 Overview of Abstraction.
15.2 Overview of Inheritance.
15.3 Overview of Encapsulation.
15.4 Overview of Polymorphism.
16.Inheritance.
16.1 Class Inheritance.
16.2 Inheriting variables and Methods.
16.3 Invoking the Parent’s class method.
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
Java Back End Developer Project Life Cycle.
Phase 1: Requirement Analysis & System Design
Understand project requirements, business logic, and system architecture. Design database schemas, API endpoints, and system flow using UML diagrams or microservices architecture. Choose frameworks like Spring Boot for backend development.
Phase 2: Development & Testing
Implement backend logic using Java, integrating databases (MySQL, PostgreSQL) and APIs. Secure the application with authentication (JWT, OAuth). Perform unit, integration, and performance testing using JUnit, Mockito, and Postman.
Phase 3: Deployment & Maintenance
Deploy the backend on cloud platforms (AWS, Azure) or containers (Docker, Kubernetes). Set up CI/CD pipelines for automated deployment. Monitor logs, optimize performance, and handle bug fixes for continuous improvement.